Entries tagged "Web Development"
Rename Files on the Fly While Transferring with WinSCP
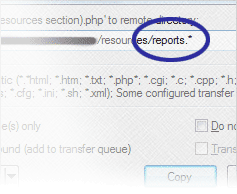
When uploading files to a website, it's easy to let WinSCP do its thing. The file name will likely be the same as the one online when fixing typos and updating content. However, what happens if the file names don't match? We'll need to upload the new file, remove the old one, and rename the new file so that it works with the website's links. The process is tedious; especially if the process is repeated dozens of times. Luckily, WinSCP provides a better way. [Continue reading]
Avoid MySQL Queries Within Loops
One consistent piece of advice that's given on PHP help forums is that queries shouldn't be executed within loops. However, they don't usually provide an alternate means for accomplishing the task at hand. So I wanted to share a solution which fixed one of my scenarios. [Continue reading]
Avoid Broken Website Links by Eliminating Whitespace in GET Variables
When naming pages for a website, its best practice to avoid spaces. Well the same goes for the GET variables (and their values) passed via the URL. Website addresses will likely work with or without spaces. However, that doesn't mean they will be free from problems. Visitors may copy a website address into an e-mail message, for example, and those spaces prevent e-mail clients like Microsoft Outlook from converting the address into a clickable link. At least a clickable link that works. [Continue reading]
Prevent Duplicate Items from Being Added to a Shopping Cart with the Header Redirect
For the longest time, I've been ignoring header redirects. They typically seem to be used for processing form requests. One page collects the form data and if everything checks out, the script redirects visitors to a confirmation page. Otherwise, the visitor is redirected back to the form. This is a perfectly acceptable way to build forms, but my preference has been to keep everything under the same page. Using a series of if constructs makes this possible. Lately, however, the header redirect has been sneaking into my scripts. In case I'm not the only one shying away from the header() function, let's talk about one possibility. [Continue reading]
WinSCP Logging into the Wrong Website Folder
In a newer version of WinSCP, a setting was added to remember the last folder used. The setting usually works as expected, but there's been a few times where I'm dropped into an inaccessible folder with no apparent way to my website's files. Restarting WinSCP has fixed this type of issue in the past. This last week, however, restarting did not work. So it's time to investigate and share the findings. [Continue reading]
Remove White Space from Form Data to Avoid Potential Headaches
When processing form submissions, it's important to remove white space before and after each value. Those extra spaces will likely go unnoticed by most visitors, but luckily this won't be a problem most of the time. In some cases, however, those spaces can lead to a lot of confusion. [Continue reading]
Deactivate Code Automatically on a Test Server
When fixing a bug or adding a new feature to a website, it may be beneficial to test the changes on a development server before going live. The problem is that some aspects of the code probably shouldn't be active on the development server. For example, we probably don't Google Analytics tracking any of those visits. The code could be manually disabled during the test phase, but are we going to remember? Instead let's look into automating the process. [Continue reading]
Future Proofing Our Code
Due to all the changes that PHP has going through over the last few years, I've spent a lot of time rethinking my coding practices. Functions such as ereg_replace() have been depreciated and eventually we won't be able to use functions like mysql_query(). With these types of changes, who knows what's safe. Let's look at what can be done to future proof our code. [Continue reading]
Reuse GET and POST Instead of Duplicating Variables
When reading data from the GET or POST array, why do we assign the information to another variable? Why can't we just use the GET or POST variables? It's not like we unset those variables after the new ones are created. Instead of duplicating variables, consider keeping the ones created for us by PHP. [Continue reading]
Why Does the fetch_array() Function Even Exist?
When looping through a MySQL result set, which function do you use? My preference has always been for the fetch_array() function since it allows access to the data using the database column names. However, is that the most efficient function to use? [Continue reading]