Alternate Way to Write PHP Function Arguments Part 3: Manually Throwing Errors
Using associative arrays for function arguments allows for more flexibility. However, we do lose some important features when it comes to building and calling functions. Packing all those arguments into an associative array prevents PHP from automatically detecting when required arguments are not included. But, there is a way to simulate this feature.
Background
In the previous post (Alternate Way to Write PHP Function Arguments Part 2: Using Associative Arrays), we built a function which displays / returns some staff member information. To control the function, a single associative array containing all the function arguments is passed. Here is the final code:
<?php
//CONNECT WITH DATABASE
require "{$_SERVER['DOCUMENT_ROOT']}/../database_connection.php";
$connect = new connect();
$mysqli = $connect->databaseObject;
//FUNCTION DISPLAYS/GETS THE INDICATED STAFF MEMBERS
function getStaff($argumentsArray=array()) {
//IF NOT PASSED, SET DEFAULT FOR THE "display" ARGUMENT
if(!isset($argumentsArray['display'])) {
$argumentsArray['display'] = true;
}
//PREPARE THE WHERE CLAUSE
$whereClause = '';
$whereOptions = array();
if(!empty($argumentsArray['types'])) { $whereOptions[] = "type in ('" . implode("', '", $argumentsArray['types']) . "')"; }
if(!empty($argumentsArray['specificIDs'])) { $whereOptions[] = "id in ('" . implode("', '", $argumentsArray['specificIDs']) . "')"; }
if(!empty($whereOptions)) { $whereClause = "WHERE " . implode(' AND ', $whereOptions); }
//GET STAFF MEMBERS
$staffMembers = array();
$sql = "SELECT firstName, lastName FROM staffList $whereClause ORDER BY lastName, firstName";
$result = $argumentsArray['mysqli']->query($sql);
while($row = $result->fetch_assoc()) {
$staffMembers[] = $row;
}
//IF DISPLAYING THE STAFF MEMBERS
if($argumentsArray['display']) {
print '<ul>';
foreach($staffMembers as $currMember) {
print "<li>{$currMember['firstName']} {$currMember['lastName']}</li>";
}
print '</ul>';
//ELSE...RETURN ARRAY
} else {
return $staffMembers;
}
}
?>
Problem
One drawback of using an associative array is that the PHP doesn't automatically throw errors when the array doesn't contain the required argument(s). In the original code from "Alternate Way to Write PHP Function Arguments Part 1: The Standard Process," the arguments were all listed out.
<?php
//FUNCTION DISPLAYS/GETS THE INDICATED STAFF MEMBERS
function getStaff($mysqli, $types=array(), $specificIDs=array(), $display=true) {
//...
}
?>
If this function is called without the required argument ($mysqli), PHP displays the warning shown in Figure 1…assuming that all errors and warnings are being shown. Note: there are different error reporting levels and errors can be hidden.
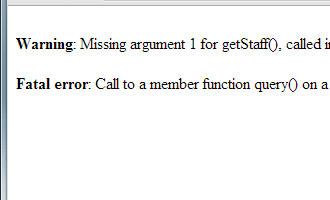
Figure 1. Missing-Argument Warning
Passing function arguments through an associative array, however, removes this type of error check. Calling the function from Part 2 without the required argument ($argumentsArray['mysqli']) doesn't lead to any warning showing up about the missing argument. You'll see an error, but that one is just saying a database connection is required to run a query.
Solution
To get PHP to throw errors for missing arguments, you can use trigger_error().
<?php
//...
//FUNCTION DISPLAYS/GETS THE INDICATED STAFF MEMBERS
function getStaff($argumentsArray=array()) {
//MAKE SURE THE REQUIRED ARGUMENTS ARE PASSED
if(!isset($argumentsArray['mysqli'])) {
trigger_error('Missing argument 1 for getStaff()', E_USER_WARNING);
}
//...
}
?>
Conclusion
The trigger_error() function gets us much closer to what we originally had before passing the function arguments through an associative array. To get even closer, you could utilize the debug_backtrace() function to determine which script called the function and what line number the function call can be found on.
0 Comments
There are currently no comments.
Leave a Comment